Automating Emails and Notifications with Python: A Comprehensive Guide
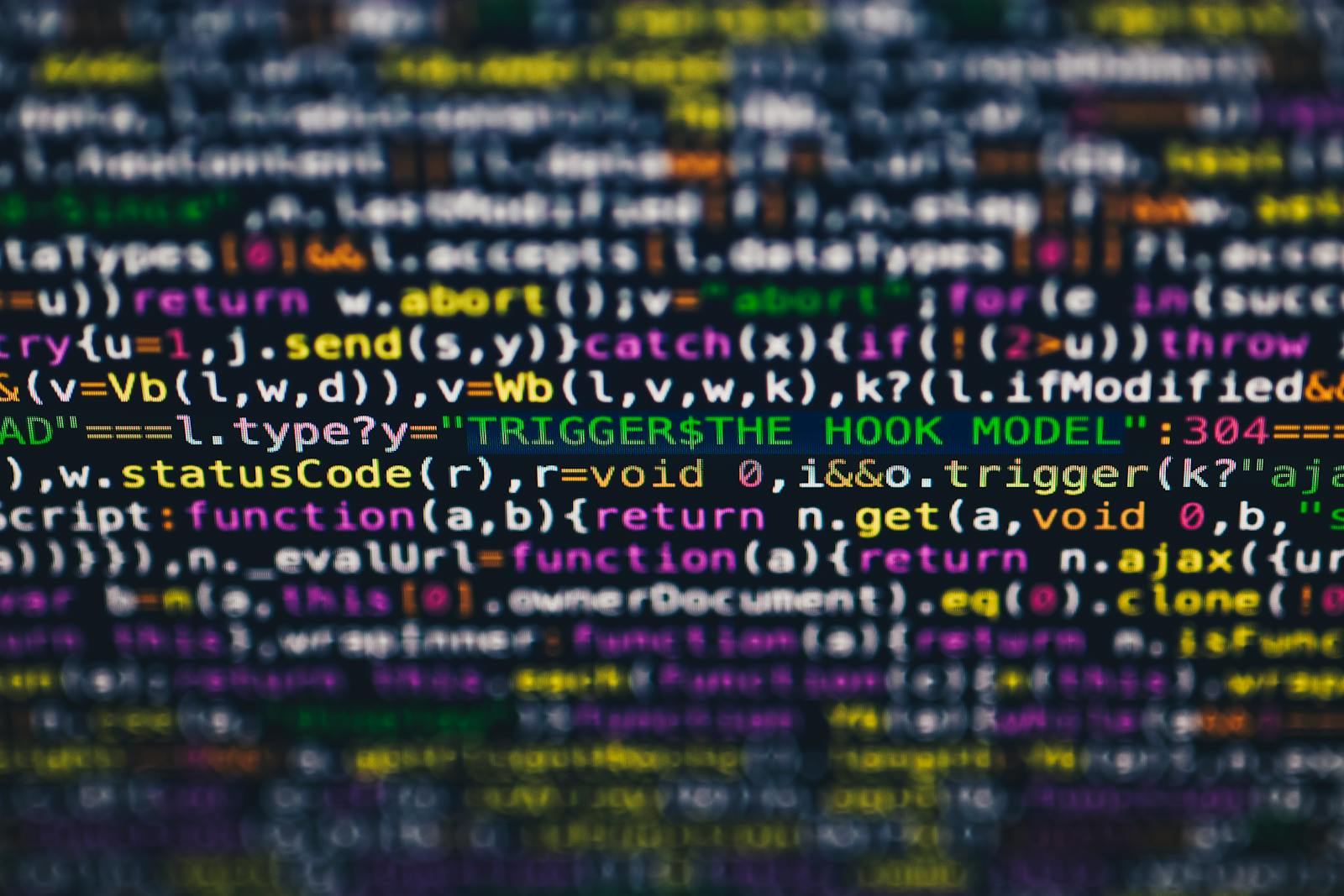
In a world bustling with digital communication, email marketing remains a stalwart strategy for businesses, from startups to industry behemoths. Now picture this: What if you could enhance those campaigns with a layer of automation, ensuring that your messages reach the right inboxes at the right time, while you focus on the finer details of your content? Enter Python, the Swiss army knife of programming, with its libraries and frameworks that can supercharge your email campaigns and alert systems, making your outreach efforts more efficient, personalized, and timely.
For Python developers, email marketers, and tech enthusiasts looking to forge stronger connections with their audiences, the art of automating emails and notifications offers a potent pathway towards engagement and growth. Stay with us as we demystify the automation process, equip you with the tools to master it, and showcase the boundless opportunities waiting at the intersection of Python and email marketing.
The Power of Email and Notification Automation
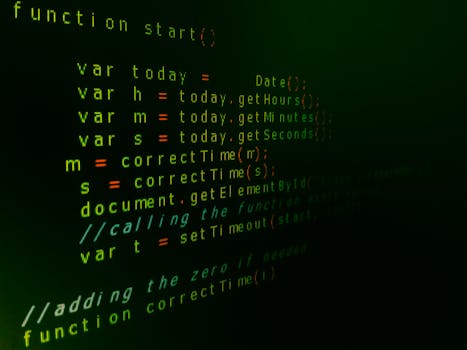
The digital marketplace moves at a breakneck pace, leaving no room for manual, tardy communication strategies. Automation has emerged as the linchpin of modern-day outreach efforts, enabling businesses to set up complex, responsive communication pipelines that engage users at every step of their digital journey. Python, owing to its vibrant ecosystem of libraries and a syntax designed for readability and ease of use, is the ideal enabler of such automated systems.
From subscription confirmations and welcome emails to abandoned cart reminders and survey requests, Python’s libraries for email and notification management offer a canvas for the most sophisticated marketing workflows. But why automate? Consider the breadth of benefits:
Effortless Efficiency
Automated systems handle the legwork, letting you queue up emails or notifications for delivery and forget about manual follow-ups. With the right setup, you can reach thousands or even millions of users with a few lines of code, a feat that would daunt even the most dedicated human workforce.
Precision and Personalization
By harnessing data and rules, Python can transform mundane communications into personal engagements. Personal touches, from addressing recipients by name to tailoring content based on their past interactions, can significantly boost the effectiveness of your campaigns.
The Perfection of Consistency
Humans are error-prone; machines, less so. Automated systems ensure that your communications adhere to your brand’s voice and style guidelines, every single time. Consistency breeds trust and recognition, two pillars of a robust marketing identity.
Join us as we unlock the potential of Python in the realm of email and notification automation, and illustrate how this powerful trifecta can revolutionize your outreach strategy.
Python Primer for Email and Notification Automation
Before we leap into the depths of Python’s potential for automation, we need to arm you with the basics. Inside the virtual walls of your Python environment, several libraries await your command, each specialized for a different email or notification task. Here’s how you can get started.
Libraries Galore
At the heart of Python’s foray into emails and notifications lie its libraries. For email automation, you’ll be cozying up with ‘smtplib’ and ’email.mime’; for notifications, ‘pushbullet’ and ‘slack-sdk’ stand at the ready. These libraries offer pre-written code that handles the nitty-gritty aspects of communication, allowing you to focus on strategy and content.
Setting Sail with Python
To chart your course in the world of Python automation, you’ll need to install the necessary packages, set up a coding environment, and ensure that your sails—err, code—are seaworthy. Fear not, the Python development community is vast, and comprehensive tutorials for every skill level abound.
Get your code editor ready, your package manager willing, and your Python interpreter eager. The voyage of email and notification automation with Python awaits.
Sail Through Automated Email Seas with Python
We’re at the marina, ready to sail the sea of automated emails. With Python as your vessel, the expanse of the digital marketing ocean is yours. The ‘smtplib’ library acts as your sextant, helping to guide your emails to their final destinations, while ’email.mime’ provisions your ship with content bonanzas, from simple text messages to multimedia-rich HTML masterpieces.
Crafting and Sending Automated Emails
With Python, the simple act of sending an email transforms into an elegant piece of code:
“`
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
Set up the message
message = MIMEMultipart()
message[‘From’] = “you@your_email.com”
message[‘To’] = “[email protected]”
message[‘Subject’] = “Smooth Sailing with Python”
Add your content
message.attach(MIMEText(“Your automation odyssey begins now.”))
Connect and send
server = smtplib.SMTP(‘smtp.gmail.com’, 587)
server.starttls()
server.login(“[email protected]”, “Your Password”)
server.sendmail(“you@your_email.com”, “[email protected]”, message.as_string())
server.quit()
“`
The above abridged script demonstrates how Python can whisk away an email, personalized and pristine, to its recipient. Imagine the possibilities when you scale this process up for segmented lists, A/B testing, or time-triggered events.
Personalization at Scale
One of the Python’s formidable strengths is its ability to read, interpret, and act upon data. This feature makes it an ideal tool for personalizing automated email content, a strategy that can amplify user engagement. Dynamic content, such as personalized deals or targeted recommendations, can significantly improve the ROI of your email campaigns.
The Advanced Armada
Python equips you with tactics to handle advanced email features, such as attachments and HTML emails. An email with Python can be as informative or ornate as you desire:
“`
Adding an attachment
from email.mime.base import MIMEBase
from email import encoders
file_path=’document.pdf’
attachment = open(file_path, ‘rb’)
part = MIMEBase(‘application’, ‘octet-stream’)
part.set_payload((attachment).read())
encoders.encode_base64(part)
part.add_header(‘Content-Disposition’, “attachment; filename= ” + file_path)
message.attach(part)
“`
This snippet demonstrates how Python can smoothly attach files to your email without capsizing your intended design. The possibilities are as vast as your marketing strategy demands.
Python’s Notification Network
Emails are but one conduit for your communication with Python. The realm of notifications is a lively one, where Python excels at maintaining an agile and versatile presence across various platforms.
Pushing the Boundaries with Notifications
Push notifications, those ephemeral nudges that reside on our mobile screens, are a mainstay of modern digital interaction. In our Python-driven world, the ‘pushbullet’ library is the harbinger of these messages, allowing you to inform, entertain, and engage your audience in real-time.
“`
import pushbullet
pb = pushbullet.Pushbullet(‘YOUR API KEY’)
push = pb.push_note(“Arrival Notification”, “Your daily shipment has arrived.”)
“`
The simplicity of this code belies the depth of its impact. With Python and ‘pushbullet’ in cahoots, your notifications can be as immediate and relevant as your heart desires.
Expanding Your Reach with Other Notifications
Push notifications are just the tip of the iceberg. Python can serve notifications across various channels, thanks to libraries like ‘slack-sdk’ for the popular Slack platform or ‘twilio’ for SMS. The width of your digital moat, be it desktops, smartphones, or traditional devices, can be guarded by Python’s vigilant notifications:
“`
import slack_sdk
slack_token = “xoxb-your-token”
client = slack_sdk.WebClient(token=slack_token)
response = client.chat_postMessage(
channel=’#your-channel’,
text=”All systems operational.”
)
“`
Here, the ‘slack-sdk’ library and Python team up to ensure that your stakeholders are always informed, fostering a culture of transparency and collaboration.
Navigating the Python Postmaster Duty
Automating your email and notification strategies is akin to casting off your bot on an unmanned ship. While Python does the heavy lifting, a savvy captain knows the waters they sail.
Steering Clear of Spam
Email deliverability is a critical consideration for any marketing strategy. Sending too many emails, not authenticating your domain, or crafting spammy content can sink your deliverability rate. Python offers tools and practices to steer clear of these pitfalls, ensuring that your emails reach their intended harbors:
“`
Authenticating email with DomainKeys Identified Mail (DKIM)
message[‘DKIM-Signature’] = ‘v=1; a=rsa-sha256; c=relaxed/relaxed;’
‘d=example.com; s=mail; t=1481436601; bh=QnUM94p2aJnX…’
“`
This code snippet illustrates how Python can set up authentication for your emails, making them more trustworthy to receiving servers and, in turn, less likely to be marked as spam.
Testing the Waters
Quality assurance is the life raft for your email campaigns. Before you launch your automation armada, testing and monitoring are pivotal. Python, with its robust testing frameworks, can help you simulate real-world conditions and ensure the resilience of your automation strategies.
Automated systems aren’t immune to change, which is why monitoring the performance of your email campaigns is crucial. Python enables you to create dashboards and alerts that keep you informed of any deviations from your expected course.
Security Surveys
The high seas of the internet are teeming with threats. When you automate your email and notification processes with Python, it’s vital to fortify your systems against potential security breaches. From encrypting sensitive data to using secure connection protocols, Python’s arsenal is well-stocked to keep your communication channels safe and your audience’s trust intact.
Charting Success with Python Email and Notification Case Studies
The theoretical voyage is essential, but practical experience is the wind in your automation sails. We’ll share case studies and real-world examples of how Python has successfully navigated the realms of automated emails and notifications, proving that the investment in learning this art is more than justified.
Case Study: Retail Giant’s Personalization Powers
A retail giant harnessed Python’s might to elevate its customer engagement. Utilizing Python’s data analysis capabilities, the company segmented its customer base, tailored offers based on purchase history, and sent out beautifully crafted, HTML-rich emails that mirrored a customer’s shopping preferences. The result was a surge in open and conversion rates, indicative of the power of personalization at scale.
Case Study: Startup’s Immediacy with Push Notifications
A burgeoning tech startup leveraged Python’s prowess to orchestrate push notifications that kept their user base engaged and informed. By integrating ‘pushbullet’ with their internal systems, the startup ensured that service updates, new feature announcements, and critical information were delivered to users within seconds. This rapid and reliable connection with users solidified their loyalty and the startup’s brand reputation.
In Conclusion, Setting Sail into a World of Opportunity
Your knowledge of Python’s role in automating emails and notifications is the key to unlocking a world of opportunity for your marketing efforts. Efficiency, personalization, and consistency are the jewels in the crown of Python automation. By following this comprehensive guide, you’ve laid the foundation for a robust automation strategy that can transform how you engage with your audience.
For Python developers, email marketers, and tech enthusiasts, the endless vistas of automation strategies beckon. It’s time to set sail and explore the myriad applications of Python in your communication efforts. May your campaigns be as successful as they are elegant, and may the wind—ahem, Python—be forever at your back.
Your email and notification automation voyage begins now. Bon voyage!